- 4.2 Arrays and Matrices allocated(a) ch ek if ar y s lo t d lbound(a, dim), ubound(a,dim) low est/ h ig nd x ar y shape(a) sh ap e(d im n o) f r y.
- BLUPF90 Cheat Sheet. Simple explanation of parameter files, output files, and options for RENUMF90, BLUPF90, PREGSF90, and related programs. The format was from LaTeX Cheat Sheet. License: CC BY-NC-SA 3.0; Download: PDF (US Letter), LaTeX; Sample files. See my repository: masuday/data. Programming Modern Fortran Tutorial.
- Fortran Syntax Cheat Sheet Download
- Fortran Syntax Cheat Sheet Pdf
- Fortran Syntax Cheat Sheet Free
- Fortran Syntax Cheat Sheet Printable
Julia is an open-source, multi-platform,high-level, high-performance programming language for technicalcomputing.
Consider the Fortran 90/95 (F95 for short) programming language and syntax. Using emacs enter the following text into a file called ex1.f90, the.f90 part of the file name is the extension indicating that this is program source code written in the Fortran 90/95 language program ex1!!
Julia has an-basedcompiler that allows it to match the performance of languages such as Cand FORTRAN without the hassle of low-level code. Because the code iscompiled on the fly you can run (bits of) code in a shell or,which is part of the recommendedworkflow .
Julia is dynamically typed, provides , and is designed forparallelism and distributed computation.
Julia has a built-in package manager.
Julia has many built-in mathematical functions, including specialfunctions (e.g. Gamma), and supports complex numbers right out of thebox.
Julia allows you to generate code automagically thanks to Lisp-inspiredmacros.
Julia was born in 2012.
[designed specifically for understanding and modifying simple-dmrg]
For a programmer, the standard, online Python tutorial is quite nice. Below, we tryto mention a few things so that you can get acquainted with thesimple-dmrg
code as quickly as possible.
Python includes a few powerful internal data structures (lists,tuples, and dictionaries), and we use numpy
(numeric python) andscipy
(additional “scientific” python routines) for linearalgebra.
Basics¶
Unlike many languages where blocks are denoted by braces or specialend
statements, blocks in python are denoted by indentation level.Thus indentation and whitespace are significant in a python program.

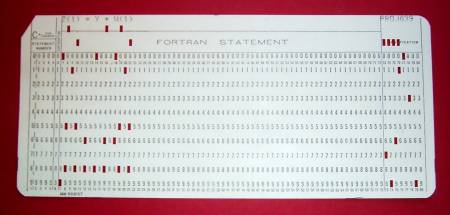
It is possible to execute python directly from the commandline:
This will bring you into python’s real-eval-print loop (REPL). Fromhere, you can experiment with various commands and expressions. Theexamples below are taken from the REPL, and include the prompts(“>>>
” and “...
”) one would see there.
Lists, tuples, and loops¶
The basic sequence data types in python are lists and tuples.
A list
can be constructed literally:
and a number of operations can be performed on it:
Note, in particular, that python uses indices counting from zero, like C (but unlike Fortran and Matlab).
A tuple
in python acts very similarly to a list, but once it is constructed it cannot be modified. It is constructed using parentheses instead of brackets:
Lists and tuples can contain any data type, and the data type of the elements need not be consistent:
It is also possible to get a subset of a list (e.g. the first threeelements) by using Python’s slice notation:
Looping over lists and tuples¶
Looping over a list
or tuple
is quite straightforward:
If you wish to have the corresponding indices for each element of thelist, the enumerate()
function will provide this:
If you have two (or more) parallel arrays with the same number ofelements and you want to loop over each of them at once, use thezip()
function:
There is a syntactic shortcut for transforming a list into a new one,known as a list comprehension:
Dictionaries¶
Dictionaries are python’s powerful mapping data type. A number,string, or even a tuple can be a key, and any data type can be thecorresponding value.
Literal construction syntax:
Lookup syntax:
Modifying (or creating) elements:
The method get()
is another way to lookup an element, but returnsthe special value None
if the key does not exist (instead ofraising an error):
Looping over dictionaries¶
Looping over the keys of a dictionary:
Looping over the values of a dictionary:
Looping over the keys and values, together:
Functions¶
Function definition in python uses the def
keyword:
Function calling uses parentheses, along with any arguments to be passed:
When calling a function, it is also possibly to specify the arguments by name (e.g. x=4
):
An alternative syntax for writing a one-line function is to use python’s lambda
keyword:
numpy arrays¶
numpy
provides a multi-dimensional array type. Unlike lists andtuples, numpy
arrays have fixed size and hold values of a singledata type. This allows the program to perform operations on largearrays very quickly.
Literal construction of a 2x2 matrix:
Note that dtype='d'
specifies that the type of the array shouldbe double-precision (real) floating point.

It is also possibly to construct an array of all zeros:
And then elements can be added one-by-one:
It is possible to access a given row or column by index:
or to access multiple columns (or rows) at once:
For matrix-vector (or matrix-matrix) multiplication use thenp.dot()
function:
Warning
One tricky thing about numpy
arrays is that they do not act asmatrices by default. In fact, if you multiply two numpy
arrays, python will attempt to multiply them element-wise!
To take an inner product, you will need to take thetranspose-conjugate of the left vector yourself:
Array storage order¶
Although a numpy
array acts as a multi-dimensional object, it isactually stored in memory as a one-dimensional contiguous array.Roughly speaking, the elements can either be stored column-by-column(“column major”, or “Fortran-style”) or row-by-row (“row major”, or“C-style”). As long as we understand the underlying storage order ofan array, we can reshape it to have different dimensions. Inparticular, the logic for taking a partial trace in simple-dmrg
uses this reshaping to make the system and environment basis elementscorrespond to the rows and columns of the matrix, respectively. Then,only a simple matrix multiplication is required to find the reduceddensity matrix.
Mathematical constants¶
Fortran Syntax Cheat Sheet Download
numpy
also provides a variety of mathematical constants:
Fortran Syntax Cheat Sheet Pdf
Experimentation and getting help¶
As mentioned above, python’s REPL can be quite useful forexperimentation and getting familiar with the language. Another thingwe can do is to import the simple-dmrg
code directly into the REPLso that we can experiment with it directly. The line:
will execute all lines except the ones within the block that says:
Fortran Syntax Cheat Sheet Free
So if we want to use the finite system algorithm, we can (assuming oursource tree is in the PYTHONPATH
, which should typically includethe current directory):
Fortran Syntax Cheat Sheet Printable
It is also possible to get help in the REPL by using python’s built-inhelp()
function on various objects, functions, and types:
